- 版本:6.2.28
- GitHub: https://github.com/nativescript-community/ui-mapbox
- NPM: https://npmjs.net.cn/package/%40nativescript-community%2Fui-mapbox
- 下载
- 昨天:3
- 上周:63
- 上个月:670
@nativescript-community/ui-mapbox
基于矢量瓦片和OpenGL的交互式、高度可定制的地图。
目录
先决条件
你需要自己的瓦片服务器,例如由 openmaptiles.org 提供的服务器,或者Mapbox API访问令牌(他们有一个 🆓 Starter 计划!),所以请在 Mapbox 上注册https://www.mapbox.com/signup/。一旦注册,请转到你的帐户 > 应用 > 新令牌。'默认密钥令牌' 是你需要的内容。
Android
Mapbox现在需要(版本 > 8.6.6)一个API密钥来下载SDK
如果你想使用比默认的8.6.6版本更高的版本,你需要将其添加到你的 app.gradle
allprojects {
repositories {
maven {
url 'https://api.mapbox.com/downloads/v2/releases/maven'
authentication {
basic(BasicAuthentication)
}
credentials {
// Do not change the username below.
// This should always be `mapbox` (not your username).
username = 'mapbox'
// Use the secret token you stored in gradle.properties as the password
password = project.properties['MAPBOX_DOWNLOADS_TOKEN'] ?: ""
}
}
}
}
安装
从你的项目的根目录运行以下命令
ns plugin add @nativescript-community/ui-mapbox
配置
在此处添加任何其他附加配置说明。
问题
如果你在iOS构建过程中遇到与Podspec版本相关的错误,最简单的修复方法是:ns platform remove ios
和 ns platform add ios
。
在Android上,插件将此添加到 <application>
节点的 app/App_Resources/Android/AndroidManifest.xml
(插件已经尝试这样做)
<service android:name="com.mapbox.services.android.telemetry.service.TelemetryService" />
如果你遇到与 TelemetryService
相关的错误,请检查它是否存在。
使用
XML
你可以从JS或TS中实例化地图。由于地图是另一个视图组件,因此它将很好地与任何NativeScript布局协同工作。你还可以轻松地将多个地图添加到同一页面或不同页面,任何布局都可以。
一个简单的布局可能如下所示
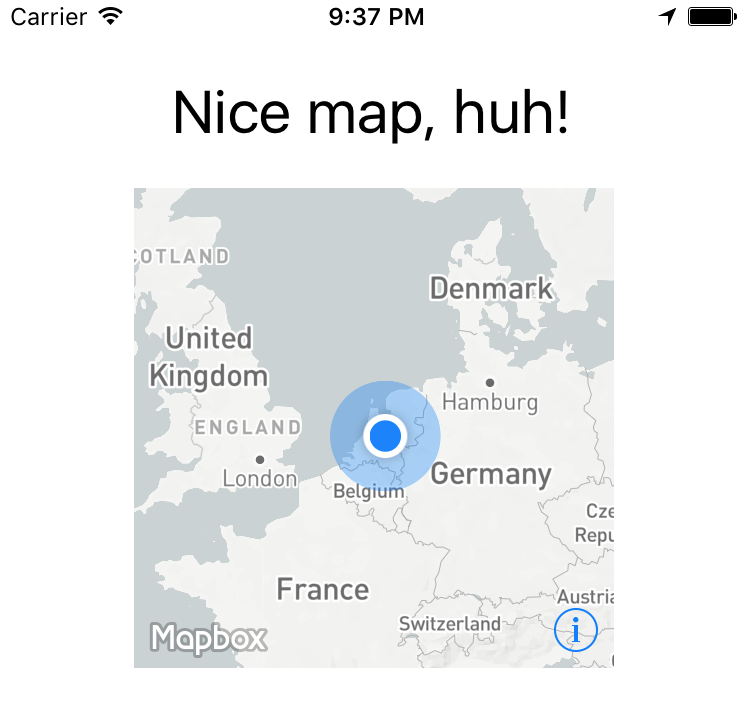
可以通过以下定义来渲染
<Page xmlns="http://schemas.nativescript.org/tns.xsd" xmlns:map="@nativescript-community/ui-mapbox" navigatingTo="navigatingTo">
<StackLayout>
<Label text="Nice map, huh!" class="title"/>
<ContentView height="240" width="240">
<map:MapboxView
accessToken="your_token"
mapStyle="traffic_night"
latitude="52.3702160"
longitude="4.8951680"
zoomLevel="3"
showUserLocation="true"
mapReady="onMapReady">
</map:MapboxView>
</ContentView>
</StackLayout>
</Page>
Angular
组件
import { registerElement } from '@nativescript/angular';
registerElement("Mapbox", () => require("@nativescript-community/ui-mapbox").MapboxView);
视图
<ContentView height="100%" width="100%">
<Mapbox
accessToken="your_token"
mapStyle="traffic_day"
latitude="50.467735"
longitude="13.427718"
hideCompass="true"
zoomLevel="18"
showUserLocation="false"
disableZoom="false"
disableRotation="false"
disableScroll="false"
disableTilt="false"
(mapReady)="onMapReady($event)">
</Mapbox>
</ContentView>
API
您基于XML的地图目前支持的所有选项为(请勿使用其他属性 - 如果您需要样式,请将地图包裹在ContentView
中,并将诸如width
之类的属性应用于该容器!)
选项 | 默认值 | 描述 |
---|---|---|
accesstoken |
- | 见上方的'先决条件'部分 |
delay |
0 | 以毫秒为单位的延迟 - 您可以设置此值以更好地控制Mapbox的调用时间,这样它就不会与您的应用程序可能需要执行的其他计算发生冲突。 |
mapStyle |
streets | streets, light, dark, satellite_streets, satellite, traffic_day, traffic_night, 以mapbox://开头的URL或指向自定义JSON定义的URL(http://, https://或相对于nativescript应用程序路径的本地相对路径 ~/) |
latitude |
- | 通过传递此值来设置地图的中心 |
longitude |
- | 以及这个 |
zoomLevel |
0 | 0-20 |
showUserLocation |
false | 在Android上需要位置权限,如果您不需要,则可以从AndroidManifest.xml 中删除 |
hideCompass |
false | 在地图旋转时不要显示指南针 |
hideLogo |
false | 如果您处于免费计划中,Mapbox要求为false |
hideAttribution |
true | 如果您处于免费计划中,Mapbox要求为false |
disableZoom |
false | 不允许用户缩放(捏合和双击) |
disableRotation |
false | 不允许用户旋转地图(双指手势) |
disableScroll |
false | 不允许用户移动地图的中心(单指拖动) |
disableTilt |
false | 不允许用户倾斜地图(双指上下拖动) |
mapReady |
- | 您可以声明的回调函数的名称,您可以在地图绘制后与之交互 |
moveBeginEvent |
- | 当地图开始移动时将被调用的函数的名称 |
moveEndEvent |
- | 当地图完成移动时将被调用的函数的名称 |
locationPermissionGranted |
- | 您可以声明的回调函数的名称,当用户授予位置权限时通知 |
locationPermissionDenied |
- | 您可以声明的回调函数的名称,当用户拒绝位置权限时通知(iOS上永远不会触发,因为没有可以拒绝的内容) |
标记
这正是上表中最后一个选项的用途 - mapReady
。它允许您在地图绘制到页面上后与之交互。
打开main-page.[js|ts]
并添加以下内容(有关完整标记API,请参阅下方的addMarkers
)
var mapbox = require("@nativescript-community/ui-mapbox");
function onMapReady(args) {
// you can tap into the native MapView objects (MGLMapView for iOS and com.mapbox.mapboxsdk.maps.MapView for Android)
var nativeMapView = args.ios ? args.ios : args.android;
console.log("Mapbox onMapReady for " + (args.ios ? "iOS" : "Android") + ", native object received: " + nativeMapView);
// .. or use the convenience methods exposed on args.map, for instance:
args.map.addMarkers([
{
lat: 52.3602160,
lng: 4.8891680,
title: 'One-line title here',
subtitle: 'Really really nice location',
selected: true, // makes the callout show immediately when the marker is added (note: only 1 marker can be selected at a time)
onCalloutTap: function(){console.log("'Nice location' marker callout tapped");}
}]
);
}
exports.onMapReady = onMapReady;
视口
var mapbox = require("@nativescript-community/ui-mapbox");
function onMapReady(args) {
args.map.setViewport(
{
bounds: {
north: 52.4820,
east: 5.1087,
south: 52.2581,
west: 4.6816
},
animated: true
}
);
}
exports.onMapReady = onMapReady;
您可以从XML声明的地图中调用以下方法:addMarkers
、setViewport
、removeMarkers
、getCenter
、setCenter
、getZoomLevel
、setZoomLevel
、getViewport
、getTilt
、setTilt
、setMapStyle
、animateCamera
、addPolygon
、removePolygons
、addPolyline
、removePolylines
、getUserLocation
、trackUser
、setOnMapClickListener
、setOnMapLongClickListener
和destroy
。
请查看下方函数的用法细节。
程序化声明
在您想要以编程方式添加地图的视图XML中添加一个容器。给它一个id。
<ContentView id="mapContainer" />
方法
显示
const contentView : ContentView = <ContentView>page.getViewById( 'mapContainer' );
const settings = {
// NOTE: passing in the container here.
container: contentView,
accessToken: ACCESS_TOKEN,
style: MapStyle.LIGHT,
margins: {
left: 18,
right: 18,
top: isIOS ? 390 : 454,
bottom: isIOS ? 50 : 8
},
center: {
lat: 52.3702160,
lng: 4.8951680
},
zoomLevel: 9, // 0 (most of the world) to 20, default 0
showUserLocation: true, // default false
hideAttribution: true, // default false
hideLogo: true, // default false
hideCompass: false, // default false
disableRotation: false, // default false
disableScroll: false, // default false
disableZoom: false, // default false
disableTilt: false, // default false
markers: [
{
id: 1,
lat: 52.3732160,
lng: 4.8941680,
title: 'Nice location',
subtitle: 'Really really nice location',
iconPath: 'res/markers/green_pin_marker.png',
onTap: () => console.log("'Nice location' marker tapped"),
onCalloutTap: () => console.log("'Nice location' marker callout tapped")
}
]
};
console.log( "main-view-model:: doShow(): creating new MapboxView." );
const mapView = new MapboxView();
// Bind some event handlers onto our newly created map view.
mapView.on( 'mapReady', ( args : any ) => {
console.log( "main-view-model: onMapReady fired." );
// this is an instance of class MapboxView
this.mapboxView = args.map;
// get a reference to the Mapbox API shim object so we can directly call its methods.
this.mapbox = this.mapboxView.getMapboxApi();
this.mapbox.setOnMapClickListener( point => {
console.log(`>> Map clicked: ${JSON.stringify(point)}`);
return true;
});
this.mapbox.setOnMapLongClickListener( point => {
console.log(`>> Map longpressed: ${JSON.stringify(point)}`);
return true;
});
this.mapbox.setOnScrollListener((point: LatLng) => {
// console.log(`>> Map scrolled`);
});
this.mapbox.setOnFlingListener(() => {
console.log(`>> Map flinged"`);
}).catch( err => console.log(err) );
});
mapView.setConfig( settings );
contentView.content = mapView;
隐藏
所有进一步的示例都假设已经引入了mapbox
。此外,所有函数都支持承诺,但我们省略了.then()
部分以简洁起见,因为它不会增加价值。
mapbox.hide();
取消隐藏
如果您之前调用了hide()
,则可以快速取消隐藏地图,而不是重新绘制它(这要慢得多,并且您会丢失视口位置等)。
mapbox.unhide();
销毁 💥
要完全清理地图,可以销毁而不是隐藏它
mapbox.destroy();
设置地图样式
在加载地图后,您可以更新地图样式
使用Mapbox Android SDK 6.1.x(用于插件版本4.1.0)时,我发现在使用此功能几秒后Android会崩溃,所以请仔细测试,并在有疑问的情况下不要使用它。
mapbox.setMapStyle(mapbox.MapStyle.DARK);
添加标记
import { MapboxMarker } from "@nativescript-community/ui-mapbox";
const firstMarker = <MapboxMarker>{ //cast as a MapboxMarker to pick up helper functions such as update()
id: 2, // can be user in 'removeMarkers()'
lat: 52.3602160, // mandatory
lng: 4.8891680, // mandatory
title: 'One-line title here', // no popup unless set
subtitle: 'Infamous subtitle!',
// icon: 'res://cool_marker', // preferred way, otherwise use:
icon: 'http(s)://website/coolimage.png', // from the internet (see the note at the bottom of this readme), or:
iconPath: '~/assets/markers/home_marker.png',
selected: true, // makes the callout show immediately when the marker is added (note: only 1 marker can be selected at a time)
onTap: marker => console.log("Marker tapped with title: '" + marker.title + "'"),
onCalloutTap: marker => alert("Marker callout tapped with title: '" + marker.title + "'")
};
mapbox.addMarkers([
firstMarker,
{
// more markers..
}
])
更新标记
插件版本4.2.0添加了更新标记选项。只需在您上面创建的MapboxMarker
引用上调用update
。您可以更新以下属性(除图标外所有属性)
firstMarker.update({
lat: 52.3622160,
lng: 4.8911680,
title: 'One-line title here (UPDATE)',
subtitle: 'Updated subtitle',
selected: true, // this will trigger the callout upon update
onTap: (marker: MapboxMarker) => console.log(`UPDATED Marker tapped with title: ${marker.title}`),
onCalloutTap: (marker: MapboxMarker) => alert(`UPDATED Marker callout tapped with title: ${marker.title}`)
})
移除标记
您可以选择不传递参数来删除所有标记,或者删除特定的标记ID(您之前指定的)。
// remove all markers
mapbox.removeMarkers();
// remove specific markers by id
mapbox.removeMarkers([1, 2]);
设置视口
如果您想例如使视口包含所有标记,可以使用此函数将边界设置为最外层标记的经纬度。
mapbox.setViewport(
{
bounds: {
north: 52.4820,
east: 5.1087,
south: 52.2581,
west: 4.6816
},
animated: true // default true
}
)
获取视口
mapbox.getViewport().then(
function(result) {
console.log("Mapbox getViewport done, result: " + JSON.stringify(result));
}
)
设置中心
mapbox.setCenter(
{
lat: 52.3602160, // mandatory
lng: 4.8891680, // mandatory
animated: false // default true
}
)
获取中心
这里使用promise回调是有意义的,所以将其添加到示例中。
mapbox.getCenter().then(
function(result) {
console.log("Mapbox getCenter done, result: " + JSON.stringify(result));
},
function(error) {
console.log("mapbox getCenter error: " + error);
}
)
设置缩放级别
mapbox.setZoomLevel(
{
level: 6.5, // mandatory, 0-20
animated: true // default true
}
)
获取缩放级别
mapbox.getZoomLevel().then(
function(result) {
console.log("Mapbox getZoomLevel done, result: " + JSON.stringify(result));
},
function(error) {
console.log("mapbox getZoomLevel error: " + error);
}
)
动画相机
// this is a boring triangle drawn near Amsterdam Central Station
mapbox.animateCamera({
// this is where we animate to
target: {
lat: 52.3732160,
lng: 4.8941680
},
zoomLevel: 17, // Android
altitude: 2000, // iOS (meters from the ground)
bearing: 270, // Where the camera is pointing, 0-360 (degrees)
tilt: 50,
duration: 5000 // default 10000 (milliseconds)
})
设置倾斜(仅限Android)
mapbox.setTilt(
{
tilt: 40, // default 30 (degrees angle)
duration: 4000 // default 5000 (milliseconds)
}
)
获取倾斜(仅限Android)
mapbox.getTilt().then(
function(tilt) {
console.log("Current map tilt: " + tilt);
}
)
获取用户位置
如果用户的位置显示在地图上,您可以获取他们的坐标和速度。
mapbox.getUserLocation().then(
function(userLocation) {
console.log("Current user location: " + userLocation.location.lat + ", " + userLocation.location.lng);
console.log("Current user speed: " + userLocation.speed);
}
)
跟踪用户
如果您正在显示用户的位置,可以让地图追踪位置。地图将连续移动,与最后已知位置保持一致。
mapbox.trackUser({
mode: "FOLLOW_WITH_HEADING", // "NONE" | "FOLLOW" | "FOLLOW_WITH_HEADING" | "FOLLOW_WITH_COURSE"
animated: true
});
添加数据源
https://docs.mapbox.com/mapbox-gl-js/api/#map#addsource
支持的数据源类型
- 矢量
- GeoJson
- 栅格
将矢量添加到GeoJSON数据源中。
mapbox.addSource( id, {
type: 'vector',
url: 'url to source'
} );
-或-
mapbox.addSource( id, {
'type': 'geojson',
'data': {
"type": "Feature",
"geometry": {
"type": "LineString",
"coordinates": [ [ lng, lat ], [ lng, lat ], ..... ]
}
}
}
);
移除数据源
通过ID删除数据源
mapbox.removeSource( id );
添加图层
https://docs.mapbox.com/mapbox-gl-js/style-spec/#layers
支持图层类型
- 线
- 圆形
- 填充
- 符号
- 栅格
添加线
mapbox.addLayer({
'id': someid,
'type': 'line',
'source': {
'type': 'geojson',
'data': {
"type": "Feature",
"geometry": {
"type": "LineString",
"coordinates": [ [ lng, lat ], [ lng, lat ], ..... ]
}
}
}
},
'layout': {
'line-cap': 'round',
'line-join': 'round'
},
'paint': {
'line-color': '#ed6498',
'line-width': 5,
'line-opacity': .8,
'line-dash-array': [ 1, 1, 1, ..]
}
});
添加圆形
mapbox.addLayer({
"id": someid,
"type": 'circle',
"source": {
"type": 'geojson',
"data": {
"type": "Feature",
"geometry": {
"type": "Point",
"coordinates": [ lng, lat ]
}
}
},
"paint": {
"circle-radius": {
"stops": [
[0, 0],
[20, 8000 ]
],
"base": 2
},
'circle-opacity': 0.05,
'circle-color': '#ed6498',
'circle-stroke-width': 2,
'circle-stroke-color': '#ed6498'
}
});
数据源可以是GeoJson或矢量数据源描述,也可以是使用addSource()添加的数据源的ID。
移除图层
通过ID删除使用addLayer()添加的图层。
mapbox.removeLayer( id );
查询渲染特征
https://docs.mapbox.com/mapbox-gl-js/api/map/#map#queryrenderedfeatures 返回一个GeoJSON Feature对象数组,表示满足查询参数的可见特性。
mapbox
.queryRenderedFeatures({
point: {
lat: 52.3701494345567,
lng: 4.823684692382513,
},
layers: ['circle-with-source-object'],
filter: ['==', ['get', 'querySample'], '2'],
})
.then((result) => console.log('query rendered features', result))
查询数据源特征
https://docs.mapbox.com/mapbox-gl-js/api/map/#map#querysourcefeatures 返回一个GeoJSON Feature对象数组,表示满足查询参数的指定矢量瓦片或GeoJSON数据源中的特性。
mapbox
.querySourceFeatures('source_id', { filter: ['==', ['get', 'querySample'], '2'] })
.then((result) => console.log('query source features', result));
添加线点
动态地向线中添加一个点。
mapbox.addLinePoint( <id of line layer>, lnglat )
其中lnglat是一个包含两个点的数组,一个经度和一个纬度。
添加多边形(已弃用,请使用 addLayer() 代替)
绘制一个形状。就像我们小时候那样连点。
第一个用此函数绘制雪人的推特用户将获得一件T恤(来自@eddyverbruggen ;-))。
// after adding this, scroll to Amsterdam to see a semi-transparent red square
mapbox.addPolygon(
{
id: 1, // optional, can be used in 'removePolygons'
fillColor: new Color("red"),
fillOpacity: 0.7,
// stroke-related properties are only effective on iOS
strokeColor: new Color("green"),
strokeWidth: 8,
strokeOpacity: 0.5,
points: [
{
lat: 52.3923633970718,
lng: 4.902648925781249
},
{
lat: 52.35421556258807,
lng: 4.9308013916015625
},
{
lat: 52.353796172573944,
lng: 4.8799896240234375
},
{
lat: 52.3864966440161,
lng: 4.8621368408203125
},
{
lat: 52.3923633970718,
lng: 4.902648925781249
}
]
})
.then(result => console.log("Mapbox addPolygon done"))
.catch((error: string) => console.log("mapbox addPolygon error: " + error));
移除多边形
您可以选择不传递参数来删除所有多边形,或者删除特定的多边形ID(您之前指定的)。
// remove all polygons
mapbox.removePolygons();
// remove specific polygons by id
mapbox.removePolygons([1, 2]);
添加折线
已弃用。请使用addLayer()代替。
绘制折线。连接参数中给出的点。
// Draw a two segment line near Amsterdam Central Station
mapbox.addPolyline({
id: 1, // optional, can be used in 'removePolylines'
color: '#336699', // Set the color of the line (default black)
width: 7, // Set the width of the line (default 5)
opacity: 0.6, //Transparency / alpha, ranging 0-1. Default fully opaque (1).
points: [
{
'lat': 52.3833160, // mandatory
'lng': 4.8991780 // mandatory
},
{
'lat': 52.3834160,
'lng': 4.8991880
},
{
'lat': 52.3835160,
'lng': 4.8991980
}
]
});
移除折线
已弃用。请使用removeLayer()代替。
您可以选择不传递参数来删除所有折线,或者删除特定的折线ID(您之前指定的)。
// remove all polylines
mapbox.removePolylines();
// remove specific polylines by id
mapbox.removePolylines([1, 2]);
设置地图点击监听器
添加一个监听器以检索用户在地图上点击的位置的纬度和经度(非标记)。
mapbox.setOnMapClickListener((point: LatLng) => {
console.log("Map clicked at latitude: " + point.lat + ", longitude: " + point.lng);
});
设置地图长按监听器
添加一个监听器以检索用户在地图上长按的位置的纬度和经度(非标记)。
mapbox.setOnMapLongClickListener((point: LatLng) => {
console.log("Map longpressed at latitude: " + point.lat + ", longitude: " + point.lng);
});
设置滚动监听器
添加一个监听器以检索用户在地图上滚动到的位置的纬度和经度。
mapbox.setOnScrollListener((point?: LatLng) => {
console.log("Map scrolled to latitude: " + point.lat + ", longitude: " + point.lng);
});
离线地图
对于您想要用户预先加载的某些区域的情况,可以使用这些方法创建和删除离线区域。
重要阅读: Mapbox的离线地图文档。
下载离线区域
此示例在9、10和11个缩放级别上下载了地图样式“户外”的“阿姆斯特丹”区域。
mapbox.downloadOfflineRegion(
{
accessToken: accessToken, // required for Android in case no map has been shown yet
name: "Amsterdam", // this name can be used to delete the region later
style: mapbox.MapStyle.OUTDOORS,
minZoom: 9,
maxZoom: 11,
bounds: {
north: 52.4820,
east: 5.1087,
south: 52.2581,
west: 4.6816
},
// this function is called many times during a download, so
// use it to show an awesome progress bar!
onProgress: function (progress) {
console.log("Download progress: " + JSON.stringify(progress));
}
}
).then(
function() {
console.log("Offline region downloaded");
},
function(error) {
console.log("Download error: " + error);
}
);
高级示例:下载当前视口
使用mapbox.getViewport()
函数获取视口并下载其各种缩放级别。
// I spare you the error handling on this one..
mapbox.getViewport().then(function(viewport) {
mapbox.downloadOfflineRegion(
{
name: "LastViewport", // anything you like really
style: mapbox.MapStyle.LIGHT,
minZoom: viewport.zoomLevel,
maxZoom: viewport.zoomLevel + 2, // higher zoom level is lower to the ground
bounds: viewport.bounds,
onProgress: function (progress) {
console.log("Download %: " + progress.percentage);
}
}
);
});
列出离线区域
为了帮助您管理离线区域,有一个listOfflineRegions
函数您可以使用。然后您可以调用deleteOfflineRegion
(见下文)并传入name
来删除任何您喜欢的缓存的区域。
mapbox.listOfflineRegions({
// required for Android in case no map has been shown yet
accessToken: accessToken
}).then(
function(regions) {
console.log(JSON.stringify(JSON.stringify(regions));
},
function(error) {
console.log("Error while listing offline regions: " + error);
}
);
删除离线区域
您可以删除之前下载的区域。任何匹配name
参数的区域将被本地删除。
mapbox.deleteOfflineRegion({
name: "Amsterdam"
}).then(
function() {
console.log("Offline region deleted");
},
function(error) {
console.log("Error while deleting an offline region: " + error);
}
);
权限
hasFineLocationPermission / requestFineLocationPermission
在Android 6上,当针对API级别23+时,您需要在运行时请求权限才能显示地图上的用户位置。即使uses-permission
标签的ACCESS_FINE_LOCATION
已存在于AndroidManifest.xml
中。
您不需要在插件版本2.4.0+中这样做,因为当渲染地图时需要请求权限。
请注意,当以下情况发生时,hasFineLocationPermission
将返回true:
- 您正在iOS上运行此代码,或
- 您正在针对小于23的API级别,或
- 您正在使用Android < 6,或
- 您已授予权限。
mapbox.hasFineLocationPermission().then(
function(granted) {
// if this is 'false' you probably want to call 'requestFineLocationPermission' now
console.log("Has Location Permission? " + granted);
}
);
// if no permission was granted previously this will open a user consent screen
mapbox.requestFineLocationPermission().then(
function() {
console.log("Location permission requested");
}
);
请注意,如果传递了 showUserLocation : true
,则 show
函数也会检查权限。如果您在显示地图之前没有请求权限,而需要权限,则插件将在渲染地图时请求用户权限。
使用来自互联网的标记图像
如果您指定了 icon: 'http(s)://some-remote-image'
,则在 iOS 上您需要将域名列入白名单。通过 Google 查找 iOS ATS 的详细选项,但为了快速测试,您可以将其添加到 app/App_Resources/iOS/Info.plist
<key>NSAppTransportSecurity</key>
<dict>
<key>NSAllowsArbitraryLoads</key>
<true/>
</dict>
演示和开发
仓库设置
该仓库使用子模块。如果您没有使用 --recursive
进行克隆,则需要调用
git submodule update --init
用于安装和链接依赖项的包管理器必须是 pnpm
或 yarn
。 npm
将无法工作。
为了开发和测试:如果您使用 yarn
,则运行 yarn
;如果您使用 pnpm
,则运行 pnpm i
交互式菜单
要启动交互式菜单,请运行 npm start
(或 yarn start
或 pnpm start
)。这将列出所有常用脚本。
构建
npm run build.all
警告:似乎 yarn build.all
不会始终工作(在 node_modules/.bin
中找不到二进制文件),这就是为什么文档明确使用 npm run
演示
npm run demo.[ng|react|svelte|vue].[ios|android]
npm run demo.svelte.ios # Example
演示设置在某种程度上是特殊的,如果您想修改/添加演示,您不需要直接在 demo-[ng|react|svelte|vue]
中工作。相反,您在 demo-snippets/[ng|react|svelte|vue]
中工作。您可以从每个类型的 install.ts
开始,看看如何注册新的演示
贡献
更新仓库
您可以非常容易地更新仓库文件
首先更新子模块
npm run update
然后提交更改,然后更新公共文件
npm run sync
然后您可以运行 yarn|pnpm
,如果有任何更改,则提交更改的文件
更新README
npm run readme
更新文档
npm run doc
发布
发布完全由 lerna
处理(您可以添加 -- --bump major
来强制进行主要版本发布)只需运行
npm run publish
修改子模块
该仓库使用 https:// 子模块,这意味着您无法直接推送到子模块。一个简单的解决方案是修改 ~/.gitconfig
并添加
[url "ssh://[email protected]/"]
pushInsteadOf = https://github.com/
问题
如果您有任何问题/问题/评论,请随时创建一个问题或开始在 NativeScript 社区 Discord 中进行对话。