npm i --save nativescript-imagecropper-updated
- 版本:12.0.0
- GitHub: https://github.com/bthurlow/nativescript-imagecropper
- NPM: https://npmjs.net.cn/package/nativescript-imagecropper-updated
- 下载
- 昨天:0
- 上周:6
- 上个月:18
A {N} 图像裁剪插件
备注
iOS 8+
Android 17+
v2.0.0+ Android 库的版本已更改,因此裁剪器现在看起来不同,这是不兼容的更改
基于
TOCropViewController for iOS
uCrop for Android
安装
运行 tns plugin add nativescript-imagecropper
屏幕截图
裁剪器 UI & 最终结果(Android)
裁剪器 UI(iOS)
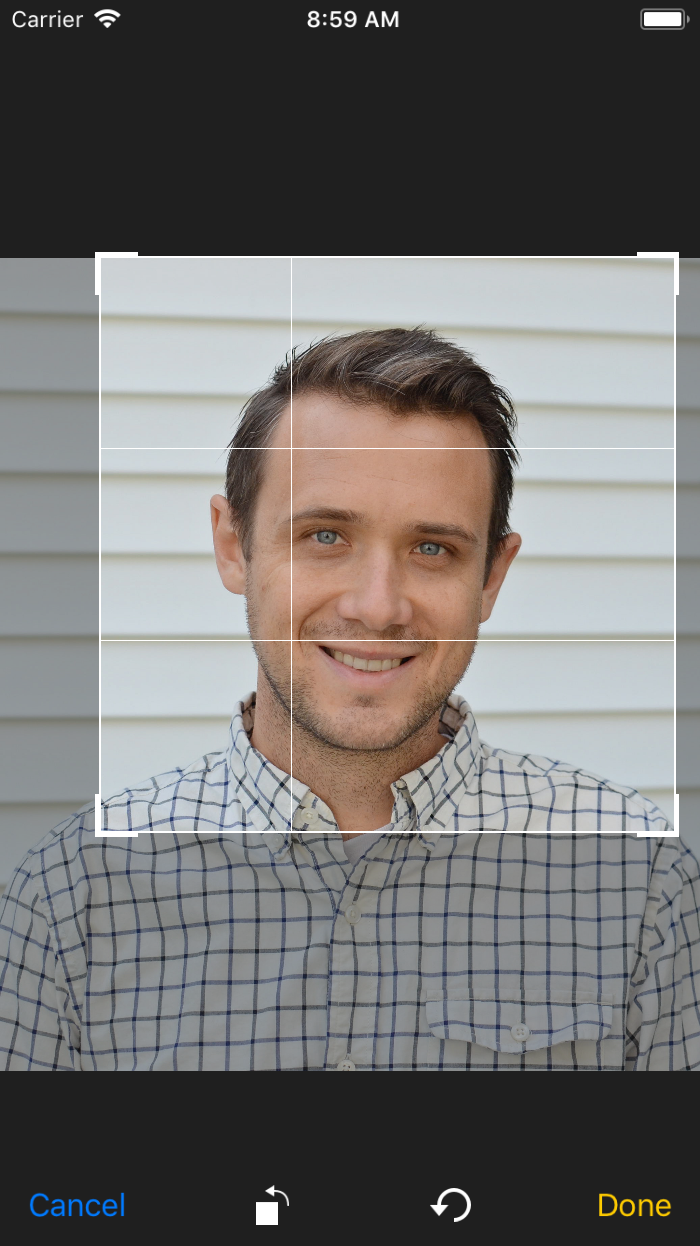
用法(请参阅示例文件夹中的示例进行 TypeScript 示例)
要使用图像裁剪模块,您必须首先引入它。
var ImageCropper = require("nativescript-imagecropper").ImageCropper;
如何获取图像源,从 nativescript-camera 插件
var camera = require("nativescript-camera");
// You might want to request camera permissions first
// check demo folder for sample implementation
camera.takePicture({width:300,height:300,keepAspectRatio:true})
.then((imageAsset) => {
let source = new imageSource.ImageSource();
source.fromAsset(imageAsset).then((source) => {
// now you have the image source
// pass it to the cropper
});
}).catch((err) => {
console.log("Error -> " + err.message);
});
方法
show(ImageSource)
:返回裁剪后的 ImageSource
var imageCropper = new ImageCropper();
imageCropper.show(imageSource).then((args) => {
console.dir(args);
if(args.image !== null){
imageView.imageSource = args.image;
}
})
.catch(function(e){
console.dir(e);
});
show(ImageSource,Options)
:返回裁剪和调整大小的 ImageSource
var imageCropper = new ImageCropper();
imageCropper.show(imageSource,{width:300,height:300}).then((args) => {
console.dir(args);
if(args.image !== null){
imageView.imageSource = args.image;
}
})
.catch(function(e){
console.dir(e);
});
选项
选项 | 类型 | 描述 |
---|---|---|
width | number | 返回图像的宽度。 |
height | number | 返回图像的高度。 |
lockSquare | boolean | 传递此选项为 true,以在 iOS 上锁定正方形纵横比,在 Android 上,此选项没有任何区别。 |
Android 的附加说明
您可以通过在 colors.xml 文件中指定相同名称的颜色来覆盖库颜色。例如
<color name="ucrop_color_toolbar">#000000</color>
这将使工具栏颜色变为黑色,如果指定在 App_Resources/Android/values/colors.xml
文件中。
Android 样式以自定义裁剪活动/样式
<!--uCrop Activity-->
<color name="ucrop_color_toolbar">#FF6E40</color>
<color name="ucrop_color_statusbar">#CC5833</color>
<color name="ucrop_color_toolbar_widget">#fff</color>
<color name="ucrop_color_widget">#000</color>
<color name="ucrop_color_widget_active">#FF6E40</color>
<color name="ucrop_color_widget_background">#fff</color>
<color name="ucrop_color_widget_text">#000</color>
<color name="ucrop_color_progress_wheel_line">#808080</color>
<color name="ucrop_color_crop_background">#000</color>
<!--Crop View-->
<color name="ucrop_color_default_crop_grid">#80ffffff</color>
<color name="ucrop_color_default_crop_frame">#ffffff</color>
<color name="ucrop_color_default_dimmed">#8c000000</color>
<color name="ucrop_color_default_logo">#4f212121</color>
返回结果参数
参数 | 类型 | 结果 |
---|---|---|
response | string | 成功 取消 错误 |
image | ImageSource | null 如果出错或取消ImageSource 成功时 |
附加功能:与 nativescript-imagepicker 6.x 一起使用的代码片段
const context = imagepickerModule.create({
mode: 'single' // allow choosing single image
});
context
.authorize()
.then(function() {
return context.present();
})
.then(function(selection) {
selection.forEach(function(selected) {
selected.getImageAsync(source => {
const selectedImgSource = imageSource.fromNativeSource(source);
imageCropper
.show(selectedImgSource, { width: 300, height: 300 })
.then(args => {
if (args.image !== null) {
// Use args.image
}
})
.catch(function(e) {
console.log(e);
});
});
});
})
.catch(function(e) {
console.log(e);
});