- 版本:2.6.2
- GitHub: https://github.com/EddyVerbruggen/nativescript-secure-storage
- NPM: https://npmjs.net.cn/package/nativescript-secure-storage
- 下载量
- 昨日:12
- 上周:66
- 上月:347
NativeScript Secure Storage 插件
安装
从命令提示符进入您的应用根目录并执行
tns plugin add nativescript-secure-storage
示例应用
想要快速入门?查看示例!否则,继续阅读。
您可以通过在项目根目录中键入 npm run demo.ios.device
来运行示例应用。
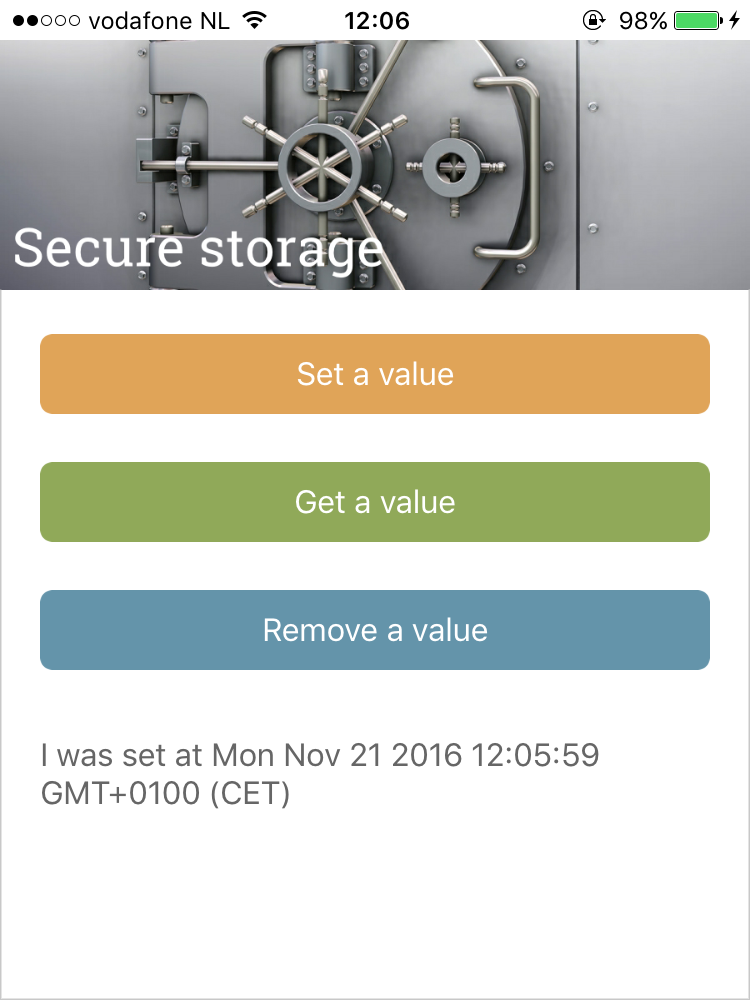
专业提示:想存储对象而不是字符串?使用 JSON.stringify
与 set
,使用 JSON.parse
与 get
。
API
set
| setSync
"为了获取某物,你首先需要设置它。"
-- Eddy Verbruggen
JavaScript
// require the plugin
var SecureStorage = require("nativescript-secure-storage").SecureStorage;
// instantiate the plugin
var secureStorage = new SecureStorage();
// async
secureStorage.set({
key: "foo",
value: "I was set at " + new Date()
}).then(
function(success) {
console.log("Successfully set a value? " + success);
}
);
// sync
var success = secureStorage.setSync({
key: "foo",
value: "I was set at " + new Date()
});
TypeScript
// require the plugin
import { SecureStorage } from "nativescript-secure-storage";
// instantiate the plugin
let secureStorage = new SecureStorage();
// async
secureStorage.set({
key: "foo",
value: "I was set at " + new Date()
}).then(success => console.log("Successfully set a value? " + success));
// sync
const success = secureStorage.setSync({
key: "foo",
value: "I was set at " + new Date()
});
get
| getSync
如果找不到,将返回 null
。
JavaScript
// async
secureStorage.get({
key: "foo"
}).then(
function(value) {
console.log("Got value: " + value);
}
);
// sync
var value = secureStorage.getSync({
key: "foo"
});
TypeScript
// async
secureStorage.get({
key: "foo"
}).then(value => console.log("Got value: " + value));
// sync
const value = secureStorage.getSync({
key: "foo"
});
remove
| removeSync
JavaScript
// async
secureStorage.remove({
key: "foo"
}).then(
function(success) {
console.log("Removed value? " + success);
}
);
// sync
var success = secureStorage.removeSync({
key: "foo"
});
TypeScript
// async
secureStorage.remove({
key: "foo"
}).then(success => console.log("Successfully removed a value? " + success));
// sync
const success = secureStorage.removeSync({
key: "foo"
});
removeAll
| removeAllSync
JavaScript
// async
secureStorage.removeAll().then(
function(success) {
console.log("Removed value? " + success);
}
);
// sync
var success = secureStorage.removeAllSync();
TypeScript
// async
secureStorage.removeAll().then(success => console.log("Successfully removed a value? " + success));
// sync
const success = secureStorage.removeAllSync();
clearAllOnFirstRun
| clearAllOnFirstRunSync
如果您想在新安装应用时清除数据,可以使用这些函数。
这仅在 iOS 上真正有用,因为如果您通过此插件向密钥链写入数据,当应用被卸载时,这些数据 不会 被删除。因此,下次安装相同的应用时,它将在密钥链中找到这些数据。
因此,如果您想从先前的安装中清除“残留”数据,确保在使用此插件提供的方法之前运行这些方法之一。
JavaScript
// async
secureStorage.clearAllOnFirstRun().then(
function(success) {
console.log(success ? "Successfully removed all data on the first run" : "Data not removed because this is not the first run");
}
);
// sync
var success = secureStorage.clearAllOnFirstRunSync();
TypeScript
// async
secureStorage.clearAllOnFirstRun().then(success => {
console.log(success ? "Successfully removed all data on the first run" : "Data not removed because this is not the first run");
});
// sync
const success = secureStorage.clearAllOnFirstRunSync();
isFirstRun
| isFirstRunSync
作为额外功能,您可以使用“首次运行”机制,当插件检测到这是首次运行(安装或安装-删除-重新安装后)时,执行任何操作。
TypeScript
// sync
if (secureStorage.isFirstRunSync()) {
// do whatever you want
}
// async
secureStorage.isFirstRun().then(isFirst => {
// if isFirst is true, do whatever you like
});
与 Angular 一起使用
在您的视图中
<Button text="set secure value" (tap)="setSecureValue()"></Button>
在您的 @Component
中
import { SecureStorage } from "nativescript-secure-storage";
export class MyComponent {
secureStorage = new SecureStorage();
// a method that can be called from your view
setSecureValue() {
this.secureStorage.set({
key: 'myKey',
value: 'my value'
}).then(success => { console.log(success)});
}
}
iOS 安全++
默认情况下,此插件使用 kSecAttrAccessibleAlwaysThisDeviceOnly
访问控制密钥链。这意味着即使设备锁定,也可以访问密钥链值。如果您想增强安全性并且不需要后台访问,或者如果您想允许值被备份并迁移到另一台设备,您可以使用此处定义的任何密钥(见此处),并在创建 SecureStorage
实例时传递它,例如
declare const kSecAttrAccessibleWhenUnlockedThisDeviceOnly; // This is needed in case you don't have tns-platform-declarations module installed.
const secureStorage = new SecureStorage(kSecAttrAccessibleWhenUnlockedThisDeviceOnly);
iOS 模拟器
目前,此插件默认在 iOS 模拟器 上使用 NSUserDefaults
。您可以通过向 SecureStorage
构造函数提供 disableFallbackToUserDefaults
来更改此行为。这样,在模拟器上使用密钥链而不是 NSUserDefaults
。
如果您遇到类似 问题_10 的问题,请考虑再次使用默认行为。
致谢
- 在 iOS 上,我们使用 SAMKeychain 库利用密钥链(在模拟器上
NSUserDefaults
), - 在 Android 上,我们使用 Hawk 库,该库内部使用 Facebook conceal。
- 感谢 Prabu Devarrajan 为 添加
deleteAll
函数!